Your first Vue.js 3 application Tutorial
In this Vue tutorial we write our first Vue application with dynamic data and styling.
We also briefly cover components, declarative rendering and reactive data.
Lesson Video
If you prefer to learn visually, you can watch this lesson in video format.
Lesson Project
This lesson is a continuation of the previous . It requires a default Vue 3 app generated by the Vue CLI .
Your first Vue application
If you expand the /src/ directory in the IDE’s Explorer pane, you’ll see a file called App.vue. Double-click the file to open it in the Code Editor.
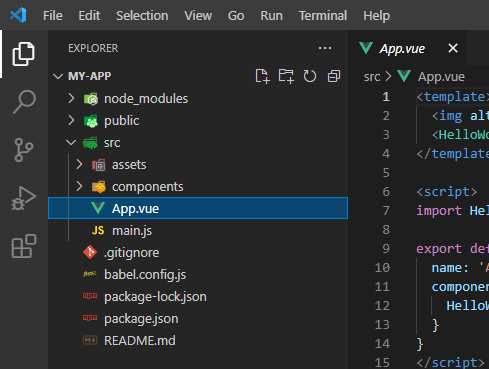
It should look something like the following.
<template>
<img alt="Vue logo" src="./assets/logo.png">
<HelloWorld msg="Welcome to Your Vue.js App"/>
</template>
<script>
import HelloWorld from './components/HelloWorld.vue'
export default {
name: 'App',
components: {
HelloWorld
}
}
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
Don’t worry about the contents for now. Remove everything in the file, then add the following code.
<template>
<h1>Hello, World!</h1>
</template>
Start the dev server with npm run serve and point your browser to localhost:8080 . You should see a big heading with the words “Hello, World!”.
Congratulations! You just made a working Vue application.
Next, we’ll make the text dynamic. That’s to say, we’ll make it depend on data that’s not hardcoded. Overwrite the previous content with the following.
<template>
<h1>Hello, {{ name }}</h1>
</template>
<script>
export default {
data() {
return { name: 'John' }
}
}
</script>
If you save the file and take a look in the browser, you should see the heading update with the words “Hello, John”.
You can add your own name between the quotes and it will update in the browser when you save the file.
Now you have a working app with dynamic data.
Finally, we’ll add some styling to the heading. Overwrite the previous content with the following.
<template>
<h1>Hello, {{ name }}</h1>
</template>
<script>
export default {
data() {
return { name: 'John' }
}
}
</script>
<style scoped>
h1 {
color: blue;
}
</style>
If you save the file and take a look in the browser, the heading should now be blue.
What you just made is a so-called component.
Components
Components are reusable units that each perform a single task. We combine those units into views (pages) that later forms a fully functional Vue application.
A component uses the .vue file extension and is made up of three language blocks.
- The template block contains the HTML markup.
- The script block contains the data and functionality for the component.
- The style block contains the styling for the markup.
<template>
<!-- HTML markup here -->
</template>
<script>
// component functionality here
</script>
<style>
/* markup styling here */
</style>
You don’t have to worry about components right now, we cover them in-depth later on in the course . For now, we’ll use the App.vue file (the root component) to learn the fundamental concepts of Vue.
Declarative rendering
Behind the scenes, Vue uses something known as declarative rendering.
It seems like we are writing HTML at first, but in reality we’re using a language that looks like HTML and allows us to extend its capabilities. The commands we write are converted and then rendered into code that the browser understands.
One thing that’s not immediately obvious from the earlier example, is that the name assigned to the heading in the template is reactive. That means that if something modifies the data in the script (like data from a database), Vue will automatically take care of modifying the HTML in template.
We don’t have to worry about modifying the HTML ourselves, we can just write commands that modify the data and Vue will take care of the rest.