TypeScript Workspace & First App Tutorial
In this TypeScript tutorial we learn how to create a workspace for our source code and write our first app.
We also discuss how to compile the TypeScript script into a Javascript script and then run that in the terminal.
Development Workspace
Before we write our first TypeScript app, we need to create a workspace. A workspace is simply a folder somewhere on our system that contains all the files that make up our TypeScript app.
- Create a single folder called ‘LearningTS’ on the Desktop.
- Open the folder as a project/workspace in your IDE.
If you’re working in Visual Studio Code, go to File > Open Folder, navigate to the ‘LearningTS’ folder on the Desktop and select Open Folder.
First app: Hello World
Now that we have a workspace, we can create the source files that will contain the code of our TypeScript app.
Inside your IDE, create a new file called ‘main.ts’.
If you’re working in Visual Studio Code, click on the New File button next to ‘LEARNINGTS’ in the File Explorer.
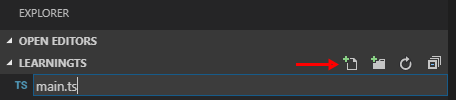
Copy and paste the following code and save the file.
function logger() {
console.log("Hello, World!");
}
logger();
How to compile a TypeScript script
Now that we have some source code, we can compile our code into something that can run on the system.
If you’re using Visual Studio Code:
VSC has an integrated terminal that we can use to compile our TypeScript scripts.
Go to Terminal > New Terminal. The terminal window will expand below the code editor and will automatically be pointed to the current folder you’re working in.
To compile and run the app, we use the command tsc followed by the file name and .ts extension.
tsc main.ts
This will compile the TypeScript script into a Javascript script. You should see a new file appear in the Project Explorer called ‘main.js’.
If you’re not using Visual Studio Code:
In Windows, click on the Start/Windows button, type cmd and press Enter to bring up the Command Prompt.
In Mac OS X, open Applications > Utilities and double-click on Terminal.
In Linux (Ubuntu / Mint), press Ctrl + Alt + T to open the terminal.
Navigate to the ‘LearningTS’ folder on your Desktop and cd into it.
cd C:\Users\YOURUSERNAME\Desktop\LearningTS
To compile and run the app we use the command tsc followed by the file name and .ts extension.
tsc main.ts
This will compile the TypeScript script into a Javascript script. You should see a new file appear in the Project Explorer called ‘main.js’.
How to run a compiled Javascript script
We can’t run TypeScript directly in the browser. We have to run the Javascript file that was generated from the TypeScript one.
In the Terminal, type the following command to run the generated Javascript file.
node main.js
You should see the words ‘Hello, World!’.
The generated .js file will typically have the same name as the original .ts file.
This two step process may be frustrating to developers at first, but you get used to it pretty quick.
Summary: Points to remember
Congratulations on writing your first TypeScript app. Granted, it’s not very exciting but it shows you just how easy TypeScript really is.