MongoDB Collection Basics Tutorial
In this tutorial we learn the basics of collections, like what they are, how to create and delete collections and how to check if a specific collection exists.
What is a Collection
A collection is simply a group of documents in MongoDB.
If you have experience with RDB’s you can think of a collection as a table.
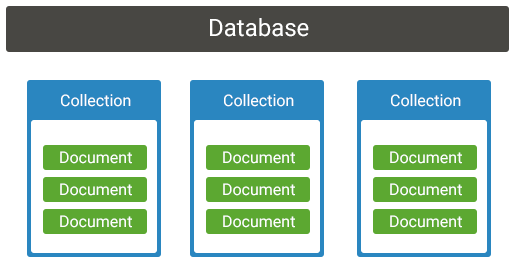
Collections do not enforce a particular schema and, typically, all documents in a collection have a similar or related purpose.
For example, if we use our normalized data model example from the Data Modelling lesson , all documents about an employee would go into an ‘employee’ collection.
Employee Collection:
- [Doc] Personal details: First and last name, and date of birth
- [Doc] Contact information: Mobile number and email address
- [Doc] Adress: Area, City, State/Province
How to create a Collection
To create a collection, we use the db.createCollection() method.
This function accepts 2 arguments.
- The first argument is the name of the collection we want to create
- The second argument allows us to specify any options about memory size and indexing
db.createCollection(name, Options)
The Options argument is a document and is completely optional. If we want to use the default options, we can simply omit the second argument.
We’ll use a database called ‘mydb’ and create a collection called ‘employee’.
Remember that strings, like names, must be wrapped in quotes.
use mydb
db.createCollection("employee")
If the collection was created, the terminal will return an OK status.
{ "ok" : 1 }
If we want to specify the options while creating a collection, we can use any of the following.
Field | Type | Description |
---|---|---|
capped | Boolean | If true, it enables a capped collection with the specified size parameter. |
A capped collection fixes its size and automatically overwrites the oldest enteries when it reaches maximum size. | ||
autoIndexID | Boolean | Deprecated since version 3.2. |
If true, it will automatically create an index on any _id fields in the documents. The default value is false. | ||
size | Number | Specifies a maximum size in bytes for a capped collection. If the capped field is true, you will need to specify this field as well. |
max | Number | Specifies the maximum number of documents allowed inside a capped collection. |
storageEngine | Document | Available for the WiredTiger storage engine only. |
Specifies the storage engine on a per-collection basis. | ||
validator | Document | Specifies the schema validation rules or expressions for a collection. |
validationLevel | String | Specifies how strictly MongoDB will apply the validator rules during an update operation. |
The available options are: | ||
“off” for no validation | ||
“strict” for validation on all insert and update operations (default) | ||
“moderate” for validation on all insert and update operations only against existing valid documents | ||
validationAction | String | Specifies whether to error on invalid documents, or just warn about violations but allow invalid documents to be inserted. |
The available options are: | ||
“error” to specify a document must pass validation before a write operation occurs, otherwise the operation will fail | ||
“warn” to specify that a warning will be logged to operation logs if the validation fails | ||
indexOptionDefaults | Document | Specifies a default configuration for indices. This option accepts a storageEngine document. |
viewOn | String | Specifies the name of the source collection or view from which to create the view. |
The name is not the full namespace of the collection or view and must be created in the same database as the source collection. | ||
pipeline | Array | Specifies an array that consists of the aggregation pipeline stage(s) . |
collation | Document | Specifies the default collation (language-specific rules for string comparison). |
The available options are: | ||
“locale” (required) | ||
“caseLevel” | ||
“caseFirst” | ||
“strength” | ||
“numericOrdering” | ||
“alternate” | ||
“maxVariable” | ||
“backwards” | ||
For a collection, you can only specify the collation during the collection creation. Once set, you cannot modify the collection’s default collation. | ||
writeConcern | Document | Specifies the document that expresses the write concern (level of acknowledgement requested from MongoDB for write operations) for the operation. |
Most methods in MongoDB have plenty of options like those above. We won’t necessarily always use all of them so we are not going to include a table of options each time in this course.
If you want to see all of a method’s options, please see the official MongoDB documentation for that specific method.
Let’s look at the simplified syntax and a small example.
db.createCollection("name",
{
"capped" : true,
"size" : num_in_bytes,
"max" : num_docs
})
db.createCollection("myCol",
{
"capped" : true,
"size" : 5000000,
"max" : 10000
})
In the example above, we create a new Collection called ‘myCol’ with the following options:
- capped:true will limit the size of the collection to the size we specify in the third key
- size:5000000 will set the maximum size of our collection to 5 million bytes, or 5 Mb
- max:10000 will allow us to store 10 thousand documents in this collection
If the operation succeeds, the terminal will return an OK status.
{ "ok" : 1 }
MongoDB will also create a collection automatically when we try to insert a value into a document.
We cover document creation and value insertion in more detail in the Document CRUD: Create lesson .
db.tutorial.insert({"name":"MongoDB Tutorial"})
The example above will create the ‘tutorial’ collection.
How to check if a Collection exists
If we want to check if a collection exists, we can use the show collections command.
show collections
This command will list all the collections in a selected database.
employee
myCol
tutorial
How to delete (drop) a Collection
If we want to delete (drop) a collection, we use the db.collection.drop() method.
db.collection_name.drop()
db.myCol.drop()
If the operation succeeds, the terminal will return a boolean true status.
true
We can confirm that the collection was deleted by running the show collections command.
employee
tutorial
Great, the ‘myCol’ collection was dropped from the database.