Javascript DOM (Document Object Model) Tutorial
In this Javascript tutorial we learn what the DOM is, how it works and how to interact with its objects and their methods and properties.
Before starting this tutorial, we recommend that you have at least the basic knowledge of objects in Javascript, which we cover in more detail in the Standalone Objects tutorial
What is the DOM
Every webpage, and the elements inside of it, can be considered to be an object. For example, the webpage itself is an object called document and has properties and methods associated with it that allows us to interact with it.
<script>
document.write("Hello Koders!");
</script>
The document object makes the write() method available to us to write some text to the webpage.
Similarly, all other document elements like <title>, <body> and <h1> are objects as well and have properties and methods available to them. All of these make up the Document Object Model, or DOM.
Objects are organized in a hierarchy. For example:
- The window object is the outermost element.
- Any html document that’s loaded into a window becomes a document object, and is a child of the window object.
The individual html elements are then children of the document object. For example:
- The html object is the outermost child of the document.
- The head and body elements are children of the html object.
- The title element is a child of the head object.
- The h1 element is a child of the body object.
Below is a simple tree to illustrate this concept.
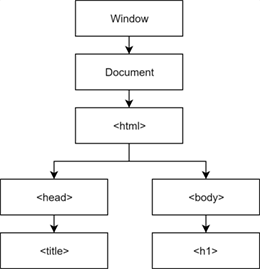
DOM Methods & Properties
We can interact with the DOM through a variety of object properties and methods.
The table below lists the properties available to DOM objects.
Property | Description |
---|---|
accessKey | Return or set the accesskey attribute of an element |
attributes | Return a NamedNodeMap of an element's attributes |
childElementCount | Return the number of child elements in an element |
childNodes | Return a collection of an element's child nodes |
children | Return a collection of an element's children |
classList | Return an element's class name(s) |
className | Return or set the value of the class attribute of an element |
clientHeight | Return the height of an element, including padding |
clientLeft | Return the width of the left border of an element |
clientTop | Return the width of the top border of an element |
clientWidth | Return the width of an element, including padding |
contentEditable | Return or set whether an element's content is editable |
dir | Return or set the value of an element's dir attribute |
id | Return or set the value of an element's id attribute |
innerHTML | Return or set the content of an element, including HTML |
innerText | Return or set the text content of a node, excluding HTML |
lang | Return or set the value of an element's lang attribute |
lastChild | Return an element's last child node |
lastElementChild | Return the last child element of a parent element |
namespaceURI | Return the namespace URI of an element |
nextSibling | Return the next node at the same node tree level |
nextElementSibling | Return the next element at the same node tree level |
nodeName | Return the name of a node |
nodeType | Return the type of a node |
nodeValue | Return or set the value of a node |
offsetHeight | Return the height of an element, including padding, border and scrollbar |
offsetWidth | Return the width of an element, including padding, border and scrollbar |
offsetLeft | Return the horizontal offset position of an element |
offsetParent | Return the offset container of an element |
offsetTop | Return the vertical offset position of an element |
outerHTML | Return or set the content of an element |
outerText | Return or set the outer text content of a node and its children |
ownerDocument | Return an element's root element (document object) |
parentNode | Return an element's parent node |
parentElement | Return an element's parent element |
previousSibling | Return the previous node at the same node tree level |
previousElementSibling | Return the previous element at the same node tree level |
scrollHeight | Return the entire height of an element, including padding |
scrollLeft | Return or set the number of pixels an element's content is scrolled horizontally |
scrollTop | Return or set the number of pixels an element's content is scrolled vertically |
scrollWidth | Return the entire width of an element, including padding |
style | Return or set the value of the style attribute of an element |
tabIndex | Return or set the value of the tabindex attribute of an element |
tagName | Return an element's tag name |
textContent | Return or set the text content of a node and its children, excluding HTML |
title | Return or set an element's title attribute |
The table below lists methods available to DOM objects.
Method | Description |
---|---|
addEventListener() | Attach an event to the specified element |
appendChild() | Add a new child node to an element as the last child node |
blur() | Remove focus from an element |
click() | Simulate a mouse-click on an element |
cloneNode() | Clone an element |
compareDocumentPosition() | Compare two elements' document position |
contains() | Return whether a node is a child of another node |
exitFullscreen() | Exit an element from fullscreen mode |
firstChild | Return an element's first child node |
firstElementChild | Return an element's first child element |
focus() | Give focus to an element |
getAttribute() | Return specified attribute value of an element |
getAttributeNode() | Return a specified attribute node |
getBoundingClientRect() | Return the size of an element and its position relative to the viewport |
getElementById() | Return an element that matches the specified id |
getElementsByName() | Return all elements that match the specified name into an array |
getElementsByTagName() | Return all elements that match the specified tag name into an array |
getElementsByClassName() | Return all elements that match the specified class into an array |
hasAttribute() | Return whether an element has the specified attribute |
hasAttributes() | Return whether an element has any attributes |
hasChildNodes() | Return whether an element has any child nodes |
insertAdjacentElement() | Insert an element at the specified position, relative to the current element |
insertAdjacentHTML() | Insert formatted text at the specified position, relative to the current element |
insertAdjacentText() | Insert text into the specified position, relative to the current element |
insertBefore() | Insert, a new child node before a specified child node |
isDefaultNamespace() | Returns whether a specified namespaceURI is the default |
isEqualNode() | Evaluate if two elements are equal |
isSameNode() | Evaluate if two elements are the same node |
isSupported() | Return whether a specified feature is supported on the element |
normalize() | Join adjacent text nodes in an element, and removes any empty text nodes |
querySelector() | Return the first child element that matches a specified CSS selector(s) |
querySelectorAll() | Return all child elements that matches a specified CSS selector(s) |
remove() | Remove an element from the DOM |
removeAttribute() | Remove a specified attribute from an element |
removeAttributeNode() | Remove a specified attribute node, and return the removed node |
removeChild() | Remove a child node from an element |
removeEventListener() | Remove an event that added with the addEventListener() method |
replaceChild() | Replace a child node in an element |
requestFullscreen() | Show an element in fullscreen mode |
scrollIntoView() | Scroll the specified element into the visible area of the browser window |
setAttribute() | Set the specified attribute to a specified value |
setAttributeNode() | Set the specified attribute node |
toString() | Convert an element to a string |
We will discuss many of these properties and methods, with examples, in the following lessons.
Summary: Points to remember
- The html document and the elements inside are objects and form the Document Object Model, or DOM.
- DOM objects have properties and methods that allow us to interact with them, accessed through dot notation.