Java Packages Tutorial
In this Java tutorial we learn how to group related classes into packages to help control access and avoid naming conflicts.
We discuss how to manually create a package in Java or how to do it in an IDE like Eclipse. Then we look at how we can use the packages and subpackages.
What are packages
A package in Java is used to group related classes together. Packages help avoid naming conflicts, control access, makes searching program elements (classes, interfaces, enums and annotations) easier and helps us maintain our code.
We can think of packages as a folder in a directory where we store related class files. The folder then becomes a package that contains the class files inside, grouped together.
A package also creates a namespace automatically, which means if two classes have the same name, but are located in different packages, the names won’t conflict with each other.
There are two types of packages in Java:
- Built-in packages, from the Java API
- User-defined packages, which we create ourselves
How to manually create a package in Java
There are several options to create a new package in Java.
If you’re not using an IDE, you will need to execute the following command in the terminal.
javac -d package_folder file_name.java
The package_folder is the folder that all the related classes, interfaces, enums and annotations will be stored.
Inside the file_name.java file, we need to mark the package in the source code with the same name as the package_folder.
To mark a package, we use the package keyword, followed by the name, at the very top of a java document.
There can only be one package definition in each source file and it applies to all types in the file.
If we don’t define a package statement, all the types in the file will be placed in the ‘default’ package.
package package_name;
A popular naming convention, and considered good practice, is to name our packages in all lowercase, to avoid any conflicts.
In this tutorial course we’re using the Eclipse IDE, which makes it easy for us to create packages.
If you’re using a different IDE, like Netbeans or IntelliJ, please refer to their documentation for the steps to follow to create a new package.
How to create a package in Eclipse IDE
To create a new package in Eclipse, go to File > New > Package.
In the New Package window, it will ask us to specify the package location and name. The location should already be populated with the directory you’re currently working on.
For the name we’ll choose ‘demo’ and click on the Finish button to create the new package. Eclipse will automatically create the appropriate directory for us.
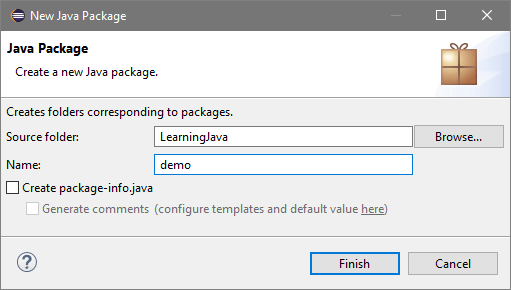
If we expand our project in the Project Explorer, we should see the new empty package.
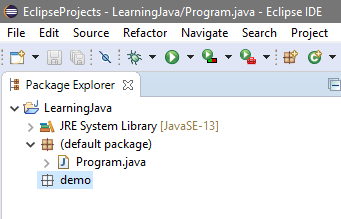
The next step is to add classes to our package. Go to File > New > Class.
In the New Class window, select ‘demo’ as the Package, name the class ‘Logger’ and click on the Finish button to create the new class.
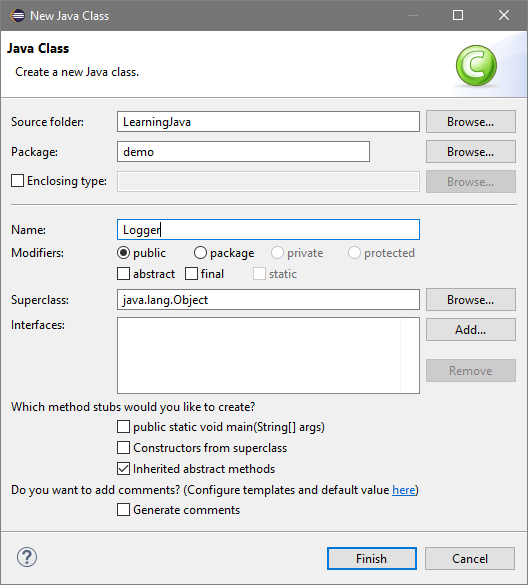
The class should open in the Code Editor window. It will have the package defined as ‘demo’ and the class defined as ‘Logger’.
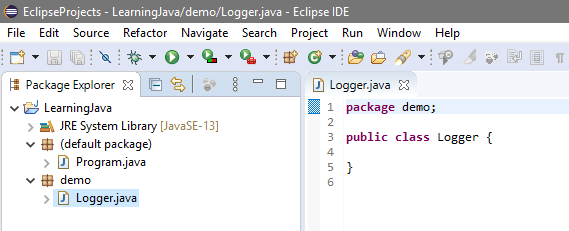
Any class that we associate with the ‘demo’ package, will be stored in its directory.
How to import a package in Java
Before we import our new package into the main program, lets add some code to the class first.
In the ‘demo’ package, in the ‘Logger’ class, add the simple method below.
package demo;
public class Logger {
public void consoleLog() {
System.out.println("Hello from the demo package");
}
}
To import the package into our main program, we use the import keyword, followed by the package name. Then, with dot notation, we access the specific class we want to import.
import package_name.class_name;
If we need to, we can import all the classes in a package with the asterisk * wildcard operator.
import package_name.*;
The asterisk after the dot operator simply means: import all from package_name.
// import 'Logger' class
// from 'demo' package
import demo.Logger;
public class Program {
public static void main(String[] args) {
// now we have 'Logger' available
// to us and we can use it like
// we normally would
Logger log = new Logger();
log.consoleLog();
}
}
The whole ‘Logger’ class is now available to us to use as we normally would.
It’s good practice to separate each class in your program into a separate class file, and group related classes into packages. It keeps your code organized and easy to find.
Subpackages in Java
We can create packages within packages to separate and organize our classes even more.
For example, we could create a package called ‘vehicles’, with subpackages for cars, trucks and motorcycles.
Typically, a company uses its domain name in reverse for packages. For example, if the company domain name is ford.com , the packages would be: com\ford
So we would end up with a directory structure like:
com\ford\vehicle\car\mustang
com\ford\vehicle\suv\everest
com\ford\vehicle\commercial\ranger
which we would reference in our IDE as packages and import them with multiple levels of dot notation.
import com.ford.vehicle.car.mustang;
import com.ford.vehicle.suv.everest;
import com.ford.vehicle.commercial.ranger;
Built-in Java API packages
Java provides us with many useful packages to help create our applications. We import and use them in the same way as custom packages.
We will go through several packages throughout the rest of the tutorial course, when and where they’re needed.
You can also see a list of all the available packages for Java 13 or the Java 13 foundation packages from the official Java documentation.
Summary: Points to remember
- A package is similar to a folder in a directory, and groups related classes, interfaces, enums and annotations together.
- To associate a class with a package, we mark the top of the document with the package keyword and its name.
- To import a class from a package, we use the import keyword and the class name with dot notation, following the directory structure.
- To import all classes from a package we can replace the class name with an asterisk wildcard.
- Java allows us to create packages within packages.
- Typically a reversed domain name is used as directory structure.
- Java provides us with many ready-made packages to use in our programs.