Go Workspace Tutorial & First App
In this Go tutorial we learn how to set up a workspace for our apps. We also write our first simple app and learn how to compile it.
Setup a Go workspace
Before we write our first Go app, we need to create a workspace. A workspace is simply a folder somewhere on our system that contains all the files that make up our Go app.
- For the sake of simplicity, we will create a single folder called ‘LearningGo’ on the Desktop.
- Open the folder as a project/workspace in your IDE.
If you’re working in Visual Studio Code, go to File > Open Folder, navigate to the ‘LearningGo’ folder on the Desktop and select Open Folder.
Go PATH
The GOPATH environment variable is where we specify the location of our workspace.
If we don’t explicitly set a GOPATH, it’s assumed to be %USERPROFILE%\go on Windows and $HOME\go on Unix systems (Mac, Linux etc.).
When we want to use a custom location for our workspace, we can explicitly set the GOPATH variable.
Windows:
Please follow the steps below to set your custom GOPATH environment variable.
- Click on the Start/Windows button, type cmd and press Enter.
- In the Command Prompt window type the following command and press Enter.
Please change the USERNAME to match yours. If you created your folder in another location, use it instead of the one below.
setx GOPATH C:\Users\USERNAME\Desktop\LearningGo
Close the Command Prompt window, the variable will be available from the next time you launch it.
Unix:
Please follow the steps below to set your custom GOPATH environment variable.
In Mac OS X, open Applications > Utilities and double-click on Terminal.
In Linux (Ubuntu / Mint), press Ctrl + Alt + T to open the terminal.
Type in the following command and press Enter.
Please change the USERNAME to match yours. If you created your folder in another location, use it instead of the one below.
go env -w GOPATH=$HOME/USERNAME/Desktop/LearningGo
First App: Hello World
Now that we have a workspace, we can create the files that will contain the code of our Go app.
Inside your IDE, create a new file called ‘main.go’.
If you’re working in Visual Studio Code, click on the New File button next to ‘LEARNINGGO’ in the File Explorer.
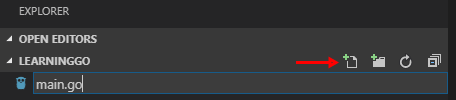
Copy and paste the following code and save the file.
package main
import "fmt"
func main() {
fmt.Println("Hello, World!")
}
How to compile a Go app
Now that we have some source code, we can compile our app into something that can run on the system.
If you’re using Visual Studio Code:
VSC has an integrated terminal that we can use to compile our Go apps.
Go to Terminal > New Terminal. The terminal window will expand below the code editor and will automatically be pointed to the current folder you’re working in.
To compile and run the app we use the command go run followed by the file name and .go extension.
go run main.go
You should see the words ‘Hello World!’ show up as output in the console.
If you’re not using Visual Studio Code:
In Windows, click on the Start/Windows button, type cmd and press Enter to bring up the Command Prompt.
In Mac OS X, open Applications > Utilities and double-click on Terminal.
In Linux (Ubuntu / Mint), press Ctrl + Alt + T to open the terminal.
Navigate to the ‘LearningGo’ folder on your Desktop and cd into it.
cd C:\Users\YOURUSERNAME\Desktop\LearningGo
To compile and run the app we use the command go run followed by the file name and .go extension.
go run main.go
You should see the words ‘Hello World!’ show up as output in the console.
Go env command
In the beginning of this tutorial, we set up a simple workspace consisting of just a folder on the desktop.
In a regular development environment we would use the console command that Go provides us to set up the workspace.
You don’t have to recreate your environment, we just want you to be aware that the console command exists.
go env
The command will create the following subfolders:
- src: contains Go source files (scripts).
Typically, this directory contains multiple version control repositories with one or more Go packages. If you use a version control system, you would create a new subdirectory inside your repository for every separate Go package.
- bin: contains the binary executables.
The Go tool will build your binary executables in this directory. All executable Go apps must contain a source file with a special package called ‘main’ which defines the entry point for the app.
- pkg: contains Go package archives (.a).
All non-executable packages (libraries) are stored in this directory. We can’t run these packages as we would binary files, they’re typically imported into executable packages.
Summary: Points to remember
Congratulations on writing your first Go app. Granted, it’s not very exciting but it shows you just how easy Go (and programming for the most part) really is.