Angular Architecture & High-level Overview
In this Angular tutorial we take a quick high-level overview of the Angular framework, it's architecture and how many of it's building blocks work.
Overview
This lesson provides a brief high-level overview of Angular’s architecture. It’s not meant as a lesson in each subject.
We cover all the concepts below in their own dedicated tutorials throughout the course, so it’s okay if you don’t understand everything at this point.
Angular Architecture
Angular’s architecture is based on the idea of coupled Components . We create individual components, that perform a single task, and put them together to form the page.
As an example, let’s consider a simple Task List application.
We have a component that is responsible for adding a task, and a component responsible for displaying tasks.
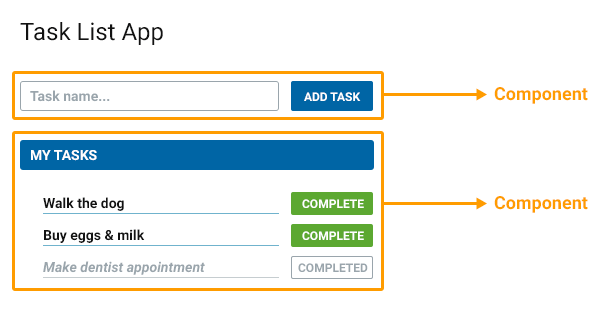
Each component is a self-contained unit of HTML, CSS and TypeScript.
The HTML and CSS is known as the View, and the Typescript is known as the Logic.
A component can be made up of other components. For example, an active task can be its own component, as well as a completed task.
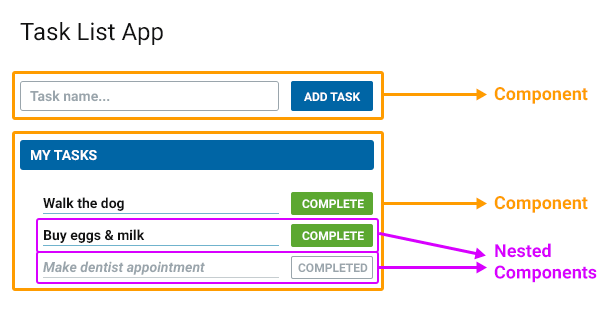
In the logic side of our components, we inject Services to define the behavior of that component. We also define Decorators to help manipulate the DOM.
Components use a process called databinding to share data between the Logic and View.
Angular Modules
An Angular application consists of several building blocks like Components, Directives and Services.
Angular groups and organizes them in a concept called Modules. The default Angular module is called the ngModule.
An application may have more than one module, but in most cases only the default one will be necessary.
Angular Modules are specific to Angular. They are not the same as Javascript Modules.
Angular Imports
Angular uses the import statement to know where to find external functionality that we use in our module.
All external dependencies like third party libraries, built-in or custom modules must be imported before we have access to their functionality.
It’s similar to the import statement from Java or the Using statement from C# .
Angular Classes and decorators
Most of the logic in Angular is built with regular TypeScript classes. We also add decorators that tell Angular how to treat a class.
For example, the @Component decorator tells Angular that the class is a component. Currently, Angular has the following decorators.
- @NgModule
- @Component
- @Injectable
- @Directive
- @Pipe
- @Input
- @Output
- @HostBinding
- @HostListener
- @ContentChild
- @ContentChildren
- @ViewChild
- @ViewChildren
Angular Building blocks
As mentioned before, an Angular application consists of several building blocks.
There are seven main building blocks.
- Components
- Templates
- Metadata
- Data Binding
- Directives
- Services
- Dependency Injection
Let’s take a quick look at all of them.
Components
A component is a TypeScript class with the @Component decorator applied to it.
A component’s View controls a part of the GUI (Graphical User Interface), and the component’s Logic controls how the component will function, both on its own and in relation to other components.
TemplatesThe template is the part of the component that defines the View.
We can either specify a location for Angular to find the HTML and CSS files that relate to the current component, or we can define the HTML and CSS inline, directly in the Decorator.
Templates consist of normal HTML and CSS code as well as Angular-specific markup, like { } for interpolation and [ ] for property binding etc.
Metadata
Metadata tells Angular how to process a class.
A class decorator uses a configuration object, with metadata, that provides Angular the information it needs about the class.
For example, the @Component decorator has metadata like selector, templateURL etc.
Data Binding
Angular uses Data Binding to communicate between a component’s View and its Logic.
This is done with special Angular-specific markup, known as Template Syntax.
Angular supports five types of Data binding:
- Interpolation binds data from the Logic to the View.
- Property Binding allows us to control the property of a HTML element.
- Event Binding is how we react to events from the HTML template.
- Two-way Binding allows us to combine Property and Event Binding to react to events and output data at the same time.
- Custom Binding allows us to communicate between parent and child components by using special decorators.
Directives
Directives are classes that help us manipulate the View.
A directive class is decorated with the @Directive decorator and contains the metadata and Logic to manipulate the DOM.
We know that Angular creates the View from the Template defined in the component. These templates are dynamic and can be transformed by Directives.
Angular supports two types of directives:
- Structural Directives can conditionally change the structure of the View.
- Attribute Directives can conditionally change the style of the View.
Angular has many built-in directives, but we are also allowed to define our own Custom Directives based on our application’s requirements.
Services
An Angular Service provides a common service or functionality to Components or other Services.
As an example, let’s consider a class that is responsible for logging messages to various parts of the application, like a failed login etc.
We don’t define logging functionality for each component, we define it as application-wide functionality, a service that can be used by any component.
Dependency Injection
Dependency Injection is the method by which we provide a component with all the resources it needs. This can be modules, services etc.
Angular does this via the Injector. It looks at the metadata, then creates an instance of the Service and injects it into the Component via its constructor.
If the service already exists, the injector won’t create it but use the existing one.
The service will have to tell Angular that it can be injected into any components that require it, by using the @Injectable decorator.